In this post, we will learn the types of Interface in C#.NET with an example in a simple way.
We have already discussed the basic concept of the interface in part one of this series. If you want to learn please click on the link basic interface concept.
Type of interface in C#
- Explicit Interface
- Implicit Interface
- Explicit Interface:If the class implement two interfaces that contain a member with the same signature.to implementing those interface member in class we take help of explicit interface.
How to implement the explicit interface?
- return_Type Interface_Name.Method_Name()
- {
- //...
- }
Note: The modifier is not valid to implement an explicit interface.
Example 1:
Create two interfaces having only one method with the same signature as follow.
- interface IVendor
- {
- void GetVendor();
- }
- interface ISupplier
- {
- void GetVendor();
- }
Create one class name like 'UserService' to implementing those interfaces.
- public class UserService:IVendor,ISupplier
- {
- void IVendor.GetVendor()
- {
- Console.WriteLine("GetVendor() method called from IVendor interface");
- }
- void ISupplier.GetVendor()
- {
- Console.WriteLine("GetVendor() method called from ISupplier interface");
- }
- }
If you try to implement the interface member without 'interface name', then it will through an error as follows.
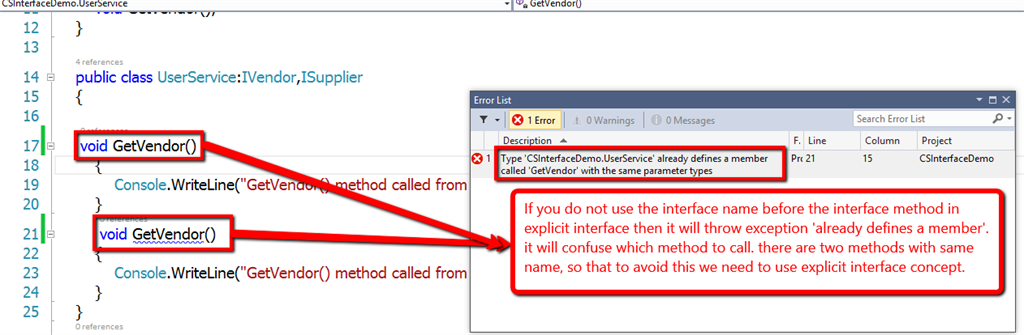
How to call the interface member?
In the explicit interface, we can not call the interface member directly using the object of the class. we have two way of calling explicit interface as following.
There is two way of calling an explicit interface member:
- The first way to creating the instance of the class and typecast the interface type.
- Second Way creating an object of the class and reference variable of the interface type.
- class Program
- {
- static void Main(string[] args)
- {
- // The first way to creating the instance of the class and typecast the interface type.
- UserService userService = new UserService();
- ((IVendor)userService).GetVendor();
- ((ISupplier)userService).GetVendor();
- // Second Way creating an object of the class and reference variable of the interface type.
- IVendor vendor = new UserService();
- vendor.GetVendor();
- ISupplier supplier = new UserService();
- supplier.GetVendor();
- Console.ReadLine();
- }
- }
Output:
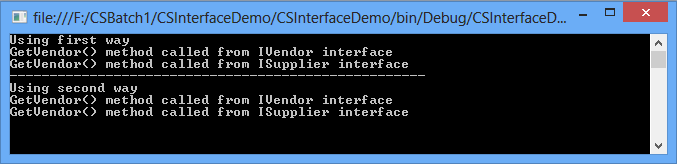
You can use either one of the ways of calling an explicit interface member as per the requirement.
Example 2:
- using System;
- namespace CSInterfaceDemo.Customer
- {
- interface IService1
- {
- void Print();
- }
- interface IService2
- {
- void Print();
- }
- public class Customer:IService1, IService2
- {
- void IService1.Print()
- {
- Console.WriteLine("IService1.Print()");
- }
- void IService2.Print()
- {
- Console.WriteLine("IService2.Print()");
- }
- }
- class program
- {
- static void Main(string[] args)
- {
- // The first way to creating the instance of the class and typecast the interface type.
- Customer customer = new Customer();
- ((IService1)customer).Print();
- ((IService2)customer).Print();
- // Second Way creating an object of the class and reference variable of the interface type.
- IService1 service1 = new Customer();
- service1.Print();
- IService2 service2 = new Customer();
- service2.Print();
- Console.ReadLine();
- }
- }
- }
2. Implicit Interface: The implicit interface does not have the same signature of the interface member. We can call the implicit interface member by using the object of the class.
Note: The modifier is required to implement the implicit interface.
How to implement the implicit interface?
- modifier return_type interface_method_name()
- {
- //....
- }
The following is an example of the implicit interface.
Example 1:
- using System;
- namespace CSInterfaceDemo.Customer
- {
- interface IService1
- {
- void Print();
- }
- interface IService2
- {
- void Display();
- }
- public class Customer:IService1, IService2
- {
- public void Print()
- {
- Console.WriteLine("IService1.Print()");
- }
- public void Display()
- {
- Console.WriteLine("IService2.Display()");
- }
- }
- class program
- {
- static void Main(string[] args)
- {
- Customer customer = new Customer();
- customer.Print();
- customer.Display();
- Console.ReadLine();
- }
- }
- }
Note :
The best practice to work with the interface by adding new code. You can achieve a new requirement by adding new separate interface instead of changing the old source code. The same advice from the Interface Segregation Principle (ISP),
The best practice to work with the interface by adding new code. You can achieve a new requirement by adding new separate interface instead of changing the old source code. The same advice from the Interface Segregation Principle (ISP),
“Clients should not be forced to depend on methods that they do not use.” by Robert C. Martin.
I hope you understood the basic concept of explicit and implicit interface.
More information watch video as follows:
More information watch video as follows:
Thanks for reading...
ReplyDeleteVery Nice Article Sir...Keep posting more Articles
ReplyDeleteThe main monetary beneficiaries are the web playing companies themselves, the overseas countries in which they're situated, and the companies that process their monetary transactions. In 1997 and 1998 a California woman named Cynthia Haines charged greater 코인카지노 than $70,000 in on-line playing losses to her bank cards. Providian National Bank, which issued the playing cards, sued her for nonpayment. In June 1998 Haines countersued the bank, claiming that it had engaged in unfair business practices by making earnings from illegal playing activities. Haines's legal professionals argued that her debt was void as a result of|as a result of} it arose from an illegal contract. Providian in the end settled out of courtroom, forgave her debt, and paid $225,000 of her attorney's fees.
ReplyDelete